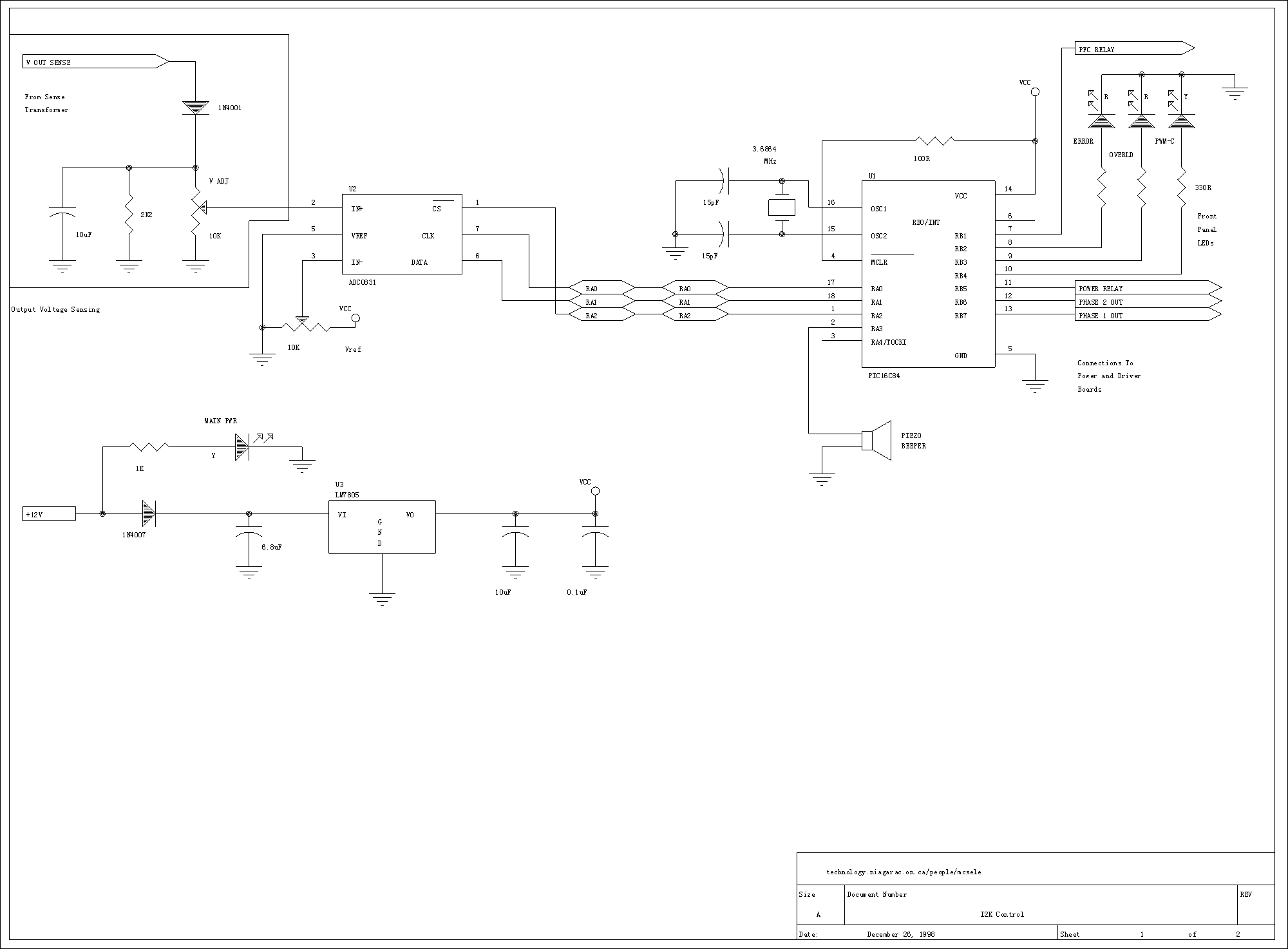
用PIC16C84的修正正弦波2kva逆变器(国外)技术资料
有兴趣的做做看
500) {this.resized=true; this.width=500; this.alt='这是一张缩略图,点击可放大。\n按住CTRL,滚动鼠标滚轮可自由缩放';this.style.cursor='hand'}" onclick="if(!this.resized) {return true;} else {window.open('http://u.dianyuan.com/bbs/u/19/1089472208.gif');}" onmousewheel="return imgzoom(this);">
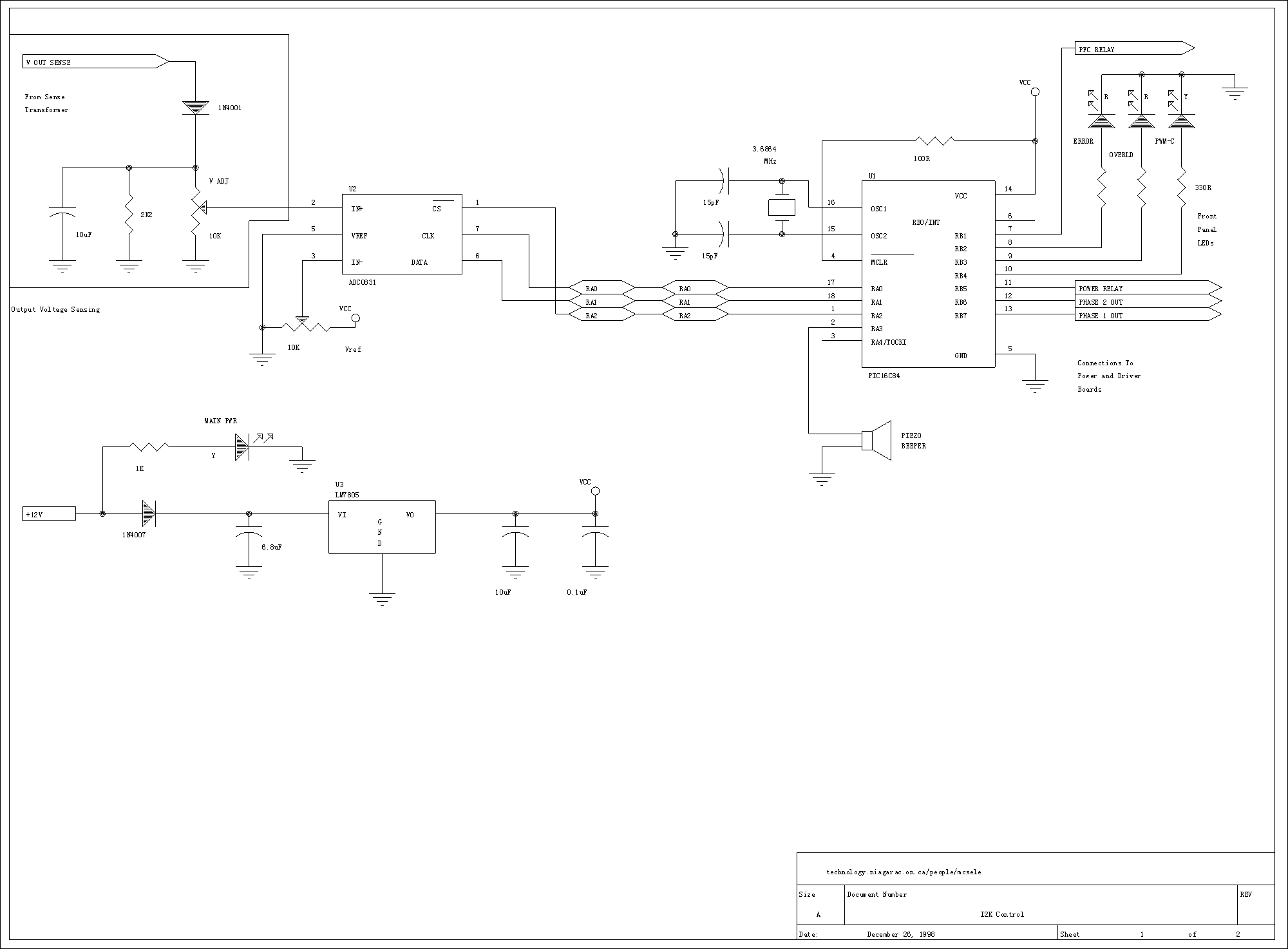
全部回复(3)
正序查看
倒序查看
现在还没有回复呢,说说你的想法
*******************主程序*******************
;*******************************************************************
;* INVERT7.ASM PIC PWM DC->AC Inverter Controller
;* Generates 60Hz PWM waveform for primary of inverter Xfrm
;* Begins at 0.5 max. width and uses ADC to sample average
;* AC output voltage. Also controls main power relays and
;* corrects load power factor
;*
;* M. Csele original version: 96/12/04 version 7: 98/12
;*
;* Hardware: Adapted for PIC Applications PCB with on-board ADC0831
;*
;* RA0 = ADC DATA Line
;* RA1 = ADC CLK Line
;* RA2 = ADC CS Line
;* RA3 = Alarm Beeper Output
;* (Used to announce startup and shutdown)
;* RA4 = [FUTURE OPTION] Shutdown Input (Active Low)
;*
;* RB0 = [FUTURE OPTION] Serial Receive/Transmit
;* RB1 = Power Factor Correction capacitor relay
;* (Switches in PF capacitor above 500VA load)
;* RB2 = Error LED Output
;* (Error code when shutdown due to fault)
;* RB3 = OverLoad LED Output
;* (Fast Blink = permissive overload)
;* (Constant = Vout < 115V at max pulse width)
;* (Slow Blink = shutdown after 6 secs of overload)
;* RB4 = PWM Change Status LED Output
;* (Lights when PW changing due to voltage error > 2)
;* RB5 = Main driver bank Relay ON Output
;* (Controls main power relays for xfmrs)
;* RB6 = Phase 2 Output to driver transistors (ACTIVE LOW)
;* RB7 = Phase 1 Output to driver transistors (ACTIVE LOW)
;*******************************************************************
LIST p=16C84 ;PIC16C84 is the target processor
INCLUDE "P16C84.INC" ;Include file with register defines
;Register Defines
Temp equ 2F ;temp storage during misc functions
ADCresult equ 2E ;stores ADC reading
ADCCtr equ 2D ;stores count for reading 8 bits from serial ADC
PulseWidth equ 2C ;Width of var. part of pulse in 10uS increments
Timer equ 2B ;Used as a count-down timer
ErrorValue equ 2A
ErrorTimerLo equ 29
ErrorTimerHi equ 28
LedBlinkTimer equ 27 ;Used for blinking LED during max. load
;PORT A Defines
ADCCLK equ 1
ADCDAT equ 0
ADCCS equ 2
BEEP equ 3
;PORT B Defines
PHASE1 equ 7
PHASE2 equ 6
PWRRELAY equ 5
LEDPWM equ 4
LEDOVERLD equ 3
LEDERROR equ 2
PFCRELAY equ 1
Main
movlw b'00000001' ;Port B all outputs except unused RB0
tris PORTB
movlw b'11000000' ;All Outputs LOW except drivers OFF (high)
movwf PORTB
movlw b'00010001' ;Port A all inputs except RA4 and RA0 (DATA)
tris PORTA
movlw b'00000000' ;All Outputs LOW
movwf PORTA
movlw .0 ;Initialize Pulse Width to 0.5 Max. (Min)
movwf PulseWidth
movlw b'10000110' ;Set timer 0 to overflow at 240Hz. 7200Hz in =
;Internal clock, Divide by 128 prescaler
bsf STATUS,RP0 ;select Page 1
movwf OPTION_REG
bcf STATUS,RP0 ;select Page 0
clrwdt
movlw .226 ;Initialize timer for 240Hz overflow
movwf TMR0
bsf PORTA,BEEP ;Beep for 1 second
movlw .240 ;1 second delay using timer
movwf Timer
NextDly
call WaitForTmr
decfsz Timer,f
goto NextDly
bcf PORTA,BEEP
bsf PORTB,PHASE1 ;Turn Phases OFF
bsf PORTB,PHASE2
bsf PORTB,PWRRELAY ;Main Power Relay ON
bcf PORTB,PFCRELAY ;Power Factor Correction OFF
;Starting Value: Minimum pulse width
movlw .1
movwf PulseWidth
;** Inverter main loop: Generate 60Hz PWMed Sine Wave and check system
MainLoop
;Phase A half-cycle output
;Generate fixed width pulse of 1/240th sec.
bcf PORTB,PHASE1 ;Phase A ON (active low)
;During Phase A fixed delay, check output voltage and adjust
;Pulse Width Accordingly for the next cycle
call ReadADC
call AdjustPulseWidth
call WaitForTmr ;Wait for 1/240th second to elapse
;Generate variable width pulse of 0 to 1/240th sec
movfw PulseWidth
movwf Timer
NextPhaseADly
call Delay10uS ;Generate from 1 to 255 10uS delays
decfsz Timer,f
goto NextPhaseADly
bsf PORTB,PHASE1 ;Phase A OFF
call WaitForTmr ;Wait for rest of 1/240th sec period
;Phase B half-cycle output
;Generate fixed width pulse of 1/240th sec.
bcf PORTB,PHASE2 ;Phase B ON (active low)
call WaitForTmr ;Wait for 1/240th second to elapse
;Generate variable width pulse of 0 to 1/240th sec
movfw PulseWidth
movwf Timer
NextPhaseBDly
call Delay10uS ;Generate from 1 to 255 10uS delays
decfsz Timer,f
goto NextPhaseBDly
bsf PORTB,PHASE2 ;Phase B OFF
call WaitForTmr ;Wait for rest of 1/240th sec period
goto MainLoop
;*******************************************************************
;* INVERT7.ASM PIC PWM DC->AC Inverter Controller
;* Generates 60Hz PWM waveform for primary of inverter Xfrm
;* Begins at 0.5 max. width and uses ADC to sample average
;* AC output voltage. Also controls main power relays and
;* corrects load power factor
;*
;* M. Csele original version: 96/12/04 version 7: 98/12
;*
;* Hardware: Adapted for PIC Applications PCB with on-board ADC0831
;*
;* RA0 = ADC DATA Line
;* RA1 = ADC CLK Line
;* RA2 = ADC CS Line
;* RA3 = Alarm Beeper Output
;* (Used to announce startup and shutdown)
;* RA4 = [FUTURE OPTION] Shutdown Input (Active Low)
;*
;* RB0 = [FUTURE OPTION] Serial Receive/Transmit
;* RB1 = Power Factor Correction capacitor relay
;* (Switches in PF capacitor above 500VA load)
;* RB2 = Error LED Output
;* (Error code when shutdown due to fault)
;* RB3 = OverLoad LED Output
;* (Fast Blink = permissive overload)
;* (Constant = Vout < 115V at max pulse width)
;* (Slow Blink = shutdown after 6 secs of overload)
;* RB4 = PWM Change Status LED Output
;* (Lights when PW changing due to voltage error > 2)
;* RB5 = Main driver bank Relay ON Output
;* (Controls main power relays for xfmrs)
;* RB6 = Phase 2 Output to driver transistors (ACTIVE LOW)
;* RB7 = Phase 1 Output to driver transistors (ACTIVE LOW)
;*******************************************************************
LIST p=16C84 ;PIC16C84 is the target processor
INCLUDE "P16C84.INC" ;Include file with register defines
;Register Defines
Temp equ 2F ;temp storage during misc functions
ADCresult equ 2E ;stores ADC reading
ADCCtr equ 2D ;stores count for reading 8 bits from serial ADC
PulseWidth equ 2C ;Width of var. part of pulse in 10uS increments
Timer equ 2B ;Used as a count-down timer
ErrorValue equ 2A
ErrorTimerLo equ 29
ErrorTimerHi equ 28
LedBlinkTimer equ 27 ;Used for blinking LED during max. load
;PORT A Defines
ADCCLK equ 1
ADCDAT equ 0
ADCCS equ 2
BEEP equ 3
;PORT B Defines
PHASE1 equ 7
PHASE2 equ 6
PWRRELAY equ 5
LEDPWM equ 4
LEDOVERLD equ 3
LEDERROR equ 2
PFCRELAY equ 1
Main
movlw b'00000001' ;Port B all outputs except unused RB0
tris PORTB
movlw b'11000000' ;All Outputs LOW except drivers OFF (high)
movwf PORTB
movlw b'00010001' ;Port A all inputs except RA4 and RA0 (DATA)
tris PORTA
movlw b'00000000' ;All Outputs LOW
movwf PORTA
movlw .0 ;Initialize Pulse Width to 0.5 Max. (Min)
movwf PulseWidth
movlw b'10000110' ;Set timer 0 to overflow at 240Hz. 7200Hz in =
;Internal clock, Divide by 128 prescaler
bsf STATUS,RP0 ;select Page 1
movwf OPTION_REG
bcf STATUS,RP0 ;select Page 0
clrwdt
movlw .226 ;Initialize timer for 240Hz overflow
movwf TMR0
bsf PORTA,BEEP ;Beep for 1 second
movlw .240 ;1 second delay using timer
movwf Timer
NextDly
call WaitForTmr
decfsz Timer,f
goto NextDly
bcf PORTA,BEEP
bsf PORTB,PHASE1 ;Turn Phases OFF
bsf PORTB,PHASE2
bsf PORTB,PWRRELAY ;Main Power Relay ON
bcf PORTB,PFCRELAY ;Power Factor Correction OFF
;Starting Value: Minimum pulse width
movlw .1
movwf PulseWidth
;** Inverter main loop: Generate 60Hz PWMed Sine Wave and check system
MainLoop
;Phase A half-cycle output
;Generate fixed width pulse of 1/240th sec.
bcf PORTB,PHASE1 ;Phase A ON (active low)
;During Phase A fixed delay, check output voltage and adjust
;Pulse Width Accordingly for the next cycle
call ReadADC
call AdjustPulseWidth
call WaitForTmr ;Wait for 1/240th second to elapse
;Generate variable width pulse of 0 to 1/240th sec
movfw PulseWidth
movwf Timer
NextPhaseADly
call Delay10uS ;Generate from 1 to 255 10uS delays
decfsz Timer,f
goto NextPhaseADly
bsf PORTB,PHASE1 ;Phase A OFF
call WaitForTmr ;Wait for rest of 1/240th sec period
;Phase B half-cycle output
;Generate fixed width pulse of 1/240th sec.
bcf PORTB,PHASE2 ;Phase B ON (active low)
call WaitForTmr ;Wait for 1/240th second to elapse
;Generate variable width pulse of 0 to 1/240th sec
movfw PulseWidth
movwf Timer
NextPhaseBDly
call Delay10uS ;Generate from 1 to 255 10uS delays
decfsz Timer,f
goto NextPhaseBDly
bsf PORTB,PHASE2 ;Phase B OFF
call WaitForTmr ;Wait for rest of 1/240th sec period
goto MainLoop
0
回复
提示
@qianyibo
*******************主程序*******************;*******************************************************************;*INVERT7.ASM PICPWMDC->ACInverterController;* Generates60HzPWMwaveformforprimaryofinverterXfrm;* Beginsat0.5max.widthandusesADCtosampleaverage;* ACoutputvoltage. Alsocontrolsmainpowerrelaysand;* correctsloadpowerfactor;*;*M.Csele originalversion:96/12/04 version7:98/12 ;*;*Hardware:AdaptedforPICApplicationsPCBwithon-boardADC0831;*;* RA0=ADCDATALine;* RA1=ADCCLKLine;* RA2=ADCCSLine;* RA3=AlarmBeeperOutput;* (Usedtoannouncestartupandshutdown) ;* RA4=[FUTUREOPTION]ShutdownInput(ActiveLow) ;*;* RB0=[FUTUREOPTION]SerialReceive/Transmit;* RB1=PowerFactorCorrectioncapacitorrelay;* (SwitchesinPFcapacitorabove500VAload);* RB2=ErrorLEDOutput;* (Errorcodewhenshutdownduetofault);* RB3=OverLoadLEDOutput;* (FastBlink=permissiveoverload);* (Constant =Vout<115Vatmaxpulsewidth);* (SlowBlink=shutdownafter6secsofoverload);* RB4=PWMChangeStatusLEDOutput;* (LightswhenPWchangingduetovoltageerror>2);* RB5=MaindriverbankRelayONOutput;* (Controlsmainpowerrelaysforxfmrs) ;* RB6=Phase2Outputtodrivertransistors(ACTIVELOW);* RB7=Phase1Outputtodrivertransistors(ACTIVELOW);******************************************************************* LIST p=16C84 ;PIC16C84isthetargetprocessor INCLUDE"P16C84.INC" ;Includefilewithregisterdefines;RegisterDefinesTemp equ 2F ;tempstorageduringmiscfunctionsADCresult equ 2E ;storesADCreadingADCCtr equ 2D ;storescountforreading8bitsfromserialADCPulseWidthequ 2C ;Widthofvar.partofpulsein10uSincrementsTimer equ 2B ;Usedasacount-downtimerErrorValue equ 2AErrorTimerLo equ 29ErrorTimerHi equ 28LedBlinkTimerequ 27 ;UsedforblinkingLEDduringmax.load ;PORTADefinesADCCLK equ 1ADCDAT equ 0ADCCS equ 2BEEP equ 3;PORTBDefinesPHASE1 equ 7PHASE2 equ 6PWRRELAY equ 5LEDPWM equ 4LEDOVERLDequ 3LEDERROR equ 2PFCRELAY equ 1Main movlw b'00000001' ;PortBalloutputsexceptunusedRB0 tris PORTB movlw b'11000000' ;AllOutputsLOWexceptdriversOFF(high) movwf PORTB movlw b'00010001' ;PortAallinputsexceptRA4andRA0(DATA) tris PORTA movlw b'00000000' ;AllOutputsLOW movwf PORTA movlw .0 ;InitializePulseWidthto0.5Max.(Min) movwf PulseWidth movlw b'10000110' ;Settimer0tooverflowat240Hz. 7200Hzin= ;Internalclock,Divideby128prescaler bsf STATUS,RP0 ;selectPage1 movwf OPTION_REG bcf STATUS,RP0 ;selectPage0 clrwdt movlw .226 ;Initializetimerfor240Hzoverflow movwf TMR0 bsf PORTA,BEEP ;Beepfor1second movlw .240 ;1seconddelayusingtimer movwf TimerNextDly call WaitForTmr decfsz Timer,f goto NextDly bcf PORTA,BEEP bsf PORTB,PHASE1 ;TurnPhasesOFF bsf PORTB,PHASE2 bsf PORTB,PWRRELAY;MainPowerRelayON bcf PORTB,PFCRELAY;PowerFactorCorrectionOFF ;StartingValue:Minimumpulsewidth movlw .1 movwf PulseWidth;**Invertermainloop:Generate60HzPWMedSineWaveandchecksystemMainLoop ;PhaseAhalf-cycleoutput ;Generatefixedwidthpulseof1/240thsec. bcf PORTB,PHASE1 ;PhaseAON(activelow) ;DuringPhaseAfixeddelay,checkoutputvoltageandadjust ;PulseWidthAccordinglyforthenextcycle call ReadADC call AdjustPulseWidth call WaitForTmr ;Waitfor1/240thsecondtoelapse ;Generatevariablewidthpulseof0to1/240thsec movfw PulseWidth movwf TimerNextPhaseADly call Delay10uS ;Generatefrom1to25510uSdelays decfsz Timer,f goto NextPhaseADly bsf PORTB,PHASE1 ;PhaseAOFF call WaitForTmr ;Waitforrestof1/240thsecperiod ;PhaseBhalf-cycleoutput ;Generatefixedwidthpulseof1/240thsec. bcf PORTB,PHASE2 ;PhaseBON(activelow) call WaitForTmr ;Waitfor1/240thsecondtoelapse ;Generatevariablewidthpulseof0to1/240thsec movfw PulseWidth movwf TimerNextPhaseBDly call Delay10uS ;Generatefrom1to25510uSdelays decfsz Timer,f goto NextPhaseBDly bsf PORTB,PHASE2 ;PhaseBOFF call WaitForTmr ;Waitforrestof1/240thsecperiod goto MainLoop
太麻烦了,我觉得用带AD的单片机来设计更简单,更重要是可以节省银子.
0
回复
提示